[Learn GO] basic knowledge
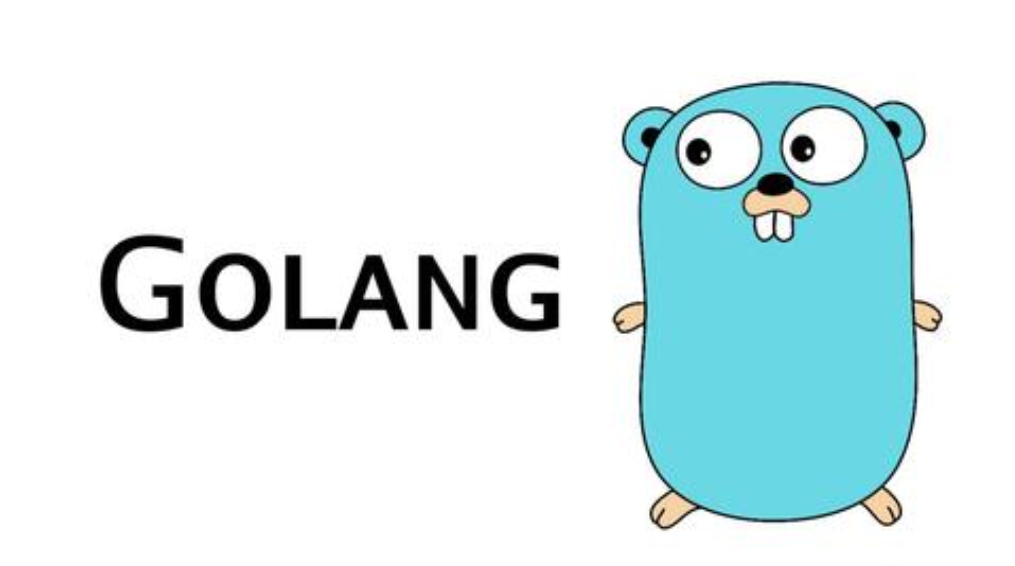
basic knowledge
- constant
- variable
- integer
- floating-point
- bool
- string
- character
- Arrays and slices
- package
basic knowledge
constant
- const
const limit = 512
const top uint16 = 1421
const Pi float64 = 3.1415926
const x,y int = 1,3
also can write in this way
const (
Cyan = 0
Black = 1
White = 2
)
true
false
iota
also default in go
itoa it reset to 0 when the const keyword appears
wa~ it's so amazing this type of const
const (
a = iota //a == 0
b = iota //b ==1
c = iota //c == 2
)
const d = iota //d==0
variable
some example:
var a int
var b string
var c float64
var d [5] int //array
var e [] int //array slice
var f * int //right
var v1 int = 5 //right
var v2 = 5 //right it can matching type automatic
v3 := 5 //right it can matching type automatic
there is a amazing code that can exchange values in this way
i := 2
j := 3
i, j = j, i //change the value, now i == 3,j == 2
int c++ or java i will do this things
int t;
t = a;
a = b;
b = a;
but in go that can in one line i,j = j,i so interesting!
interger
in go unsafe.Sizeof
it's like sizeof
int C
Go has 11 types of integer .
Talk is cheap.Show me the code.
package main
import (
"fmt"
"unsafe"
)
func main() {
a := 12
fmt.Println("length of a: ", unsafe.Sizeof(a))
var b int = 12
fmt.Println("length of b(int): ", unsafe.Sizeof(b))
var c int8 = 12
fmt.Println("length of c(int8): ", unsafe.Sizeof(c))
var d int16 = 12
fmt.Println("length of d(int16): ", unsafe.Sizeof(d))
var e int32 = 12
fmt.Println("length of e(int32): ", unsafe.Sizeof(e))
var f int64 = 12
fmt.Println("length of f(int64): ", unsafe.Sizeof(f))
}
The FMT package is used to format strings, and unsafe contains methods for getting information about the Go language type
floating-point
Go has two floating point types and two complex types
-
float 32
- The precision is about 7 decimal Numbers after the decimal point
-
float 64
- The precision is about 15 decimal Numbers after the decimal point
-
complex32
- The real and imaginary parts are all float32
-
complex64
- The real and imaginary parts are all float64
bool
- bool do not support automatic change and cast type conversion in GO
var a bool
a = true
b := (2 == 3) //b will be bool type
//error example
var b bool
b = 1 //error
b = bool(1) // error
string
t1 := "\"hello\"" //msg: "hello"
t2 := `"hello"` //msg:the same as t1
t3 := "\u6B22\u8FCE" //msg:欢迎
Go use UTF-8 its a very good way to display Chinese characters.
Strings support many operations,but as the beginner blog i don't want to write it.
that is an example
package main
import (
"fmt"
)
func main() {
t0 := "\u6B22\u8FCE\u6765\u5230" // t0内容:欢迎来到
t1 := "\u5B9E\u9A8C\u697C" // t1内容:实验楼
t2 := t0 + t1
for index, char := range t2 {
fmt.Printf("%-2d %U '%c' %X %d\n",
index, char, char, []byte(string(char)), len([]byte(string(char))))
}
fmt.Printf("length of t0: %d, t1: %d, t2: %d\n", len(t0), len(t1), len(t2))
fmt.Printf("content of t2[0:2] is: %X\n", t2[0:2])
}
here is the print on screen
$ go run string_t.go
0 U+6B22 '欢' E6ACA2 3
3 U+8FCE '迎' E8BF8E 3
6 U+6765 '来' E69DA5 3
9 U+5230 '到' E588B0 3
12 U+5B9E '实' E5AE9E 3
15 U+9A8C '验' E9AA8C 3
18 U+697C '楼' E6A5BC 3
length of t0: 12, t1: 9, t2: 21
content of t2[0:2] is: E6AC
character
Go has two character One is Byte(utf8) another is rune(Unicode )
Go use byte as usual
Arrays and slices
Go can automatically calculates the length of the array for us
[length]Type
[N]Type{value1, value2, ..., valueN}
[...]Type{value1, value2, ..., valueN}
... that is so amazing
- slices is a very good progress i think
package main
import (
"fmt"
)
func main() {
a := [...]int{1, 2, 3, 4, 5, 6, 7}
fmt.Printf("len and cap of array %v is: %d and %d\n", a, len(a), cap(a))
fmt.Printf("item in array: %v is:", a)
for _, value := range a {
fmt.Printf("% d", value)
}
fmt.Println()
s1 := a[3:6]
fmt.Printf("len and cap of slice: %v is: %d and %d\n", s1, len(s1), cap(s1))
fmt.Printf("item in slice: %v is:", s1)
for _, value := range s1 {
fmt.Printf("% d", value)
}
fmt.Println()
s1[0] = 456
fmt.Printf("item in array changed after changing slice: %v is:", s1)
for _, value := range a {
fmt.Printf("% d", value)
}
fmt.Println()
s2 := make([]int, 10, 20)
s2[4] = 5
fmt.Printf("len and cap of slice: %v is: %d and %d\n", s2, len(s2), cap(s2))
fmt.Printf("item in slice %v is:", s2)
for _, value := range s2 {
fmt.Printf("% d", value)
}
fmt.Println()
}
The print information
$ go run slice_array.go
len and cap of array [1 2 3 4 5 6 7] is: 7 and 7
item in array: [1 2 3 4 5 6 7] is: 1 2 3 4 5 6 7
len and cap of slice: [4 5 6] is: 3 and 4
item in slice: [4 5 6] is: 4 5 6
item in array changed after changing slice: [456 5 6] is: 1 2 3 456 5 6 7
len and cap of slice: [0 0 0 0 5 0 0 0 0 0] is: 10 and 20
item in slice [0 0 0 0 5 0 0 0 0 0] is: 0 0 0 0 5 0 0 0 0 0
package
Packages are collections of various types and functions
- $GOPATH
- $GOPATH's SRC directory, each package is placed in a separate directory. It can be share in all code.